Configure Tailwind CSS for a Next.js App
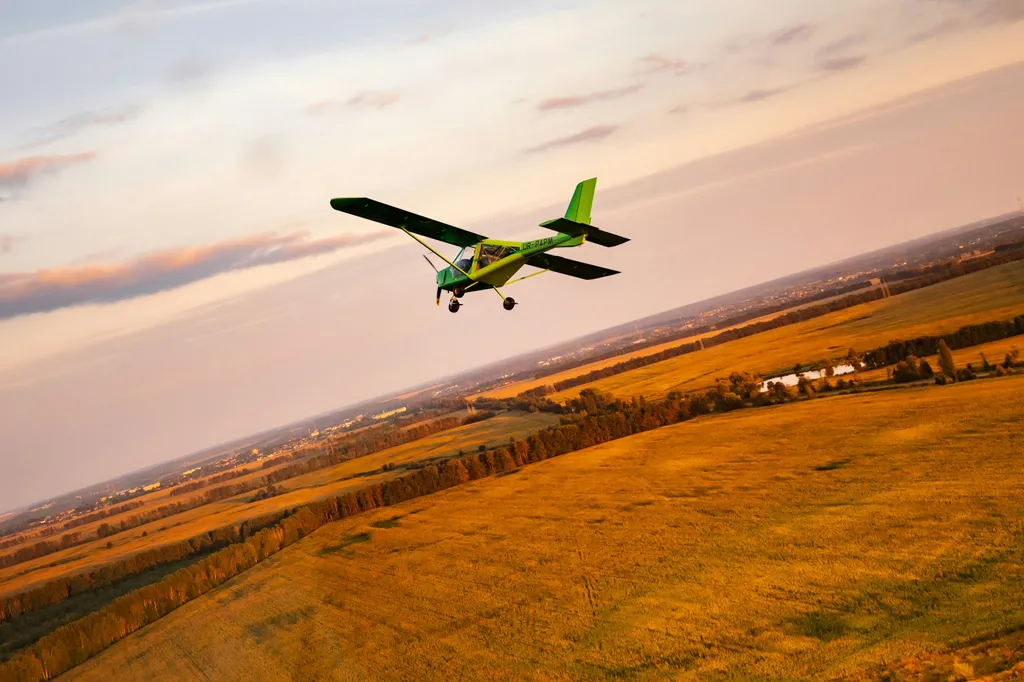
This is part 2 in the Build a Blog with Next, MDX, and Tailwind series
In this article, we’ll build on what we started in Bootstrap a Next.js Blog. We’ll get the TailwindCSS integrated into our project, add a bit of styling to verify everything works, and cleanup unnecessary CSS that came with the default project setup.
Install Dependencies
We’ll start by installing tailwindcss
, postcss
, and autoprefixer
as dev dependencies.
npm install -D tailwindcss@latest postcss@latest autoprefixer@latest
Configure Tailwind
With those dependencies installed, let’s initialize Tailwind by running Tailwind’s init
command with npx
. Passing the -p
flag will initialize tailwind along with a postcss config.
npx tailwindcss init -p
You should see a confirmation like the following
Created Tailwind CSS config file: tailwind.config.js
Created PostCSS config file: postcss.config.js
Our default tailwind.config.js
will look like this
module.exports = {
content: [],
theme: {
extend: {},
},
plugins: [],
}
In order to properly build our CSS to include only the necessary classes based on what we’ve used in our code, we’ll need to add some entries to the content
array.
We want to account for pages in the file based routing setup, any components we build, which we’ll put in the components
directory, and since we’ll be supporting MDX, we want to check the content
files for any ad-hoc styling we might apply in our MDX.
module.exports = {
- content: [],
+ content: [
+ './pages/**/*.{js,ts,jsx,tsx}',
+ './components/**/*.{js,ts,jsx,tsx}',
+ './content/**/*.md',
+ ],
theme: {
extend: {},
},
plugins: [],
}
With our config in place, let’s update the pages/_app.tsx
file to pull in the css from tailwind. We won’t be using the supplied global.css
file, so we can remove that import and add we’ll add an import from tailwind.css
from the tailwindcss
package.
-import '../styles/globals.css'
+import 'tailwindcss/tailwind.css'
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />
}
export default MyApp
With this step done, we can run the app with npm run dev
and verify that the preflight css reset that tailwind brings with it is being applied. Our “My Awesome Blog” h1
element shouldn’t be large and bold anymore.
To verify that tailwindcss styles work, let’s update the h1
in pages/index.tsx
with the following styles.
export default function Home() {
return (
<div>
<h1 className="text-sky-700 text-4xl font-black p-3">My Awesome Blog</h1>
</div>
)
}
The page should now reflect our styles from TailwindCSS.
Cleanup Unused CSS
We’ll rely on Tailwind for all our styling, so we won’t need the files that were generated when we bootstrapped our Next.js app.
We can get rid of these by deleting the whole styles
folder at the project root.
Conclusion
With Tailwind installed and configured, we’re ready to style elements as we go, using utility classes, rather than creating and managing a bunch of our own bespoke classes.
If you haven’t used Tailwind before and you’re looking at all those classnames in the h1
example above and thinking “I don’t like this!”, my advice would be to give it a fair shot. I can honestly say I’ve never been as productive in styling apps as I am with Tailwind. The utility class names are well thought out and once you start using it, it’s fairly intuitive. I frequently find myself guessing at a class before looking to the docs and I’m right more often than not because the system just makes sense.
Check back next week for the next article in the series, Apply a Common Layout to a Next.js App!