Hi!
I'm Andy. I'm a dad, a husband, an egghead.io instructor , and I'm currently working as a UI Architect building software driven networking products. I like building things for the web, and sharing what I know with others. I believe that software development is a creative endeavor. I'm passionate about code quality, testing, learning, mentoring, and training.
I share stuff on Bluesky as @vanslaars.io ,write an occasional article, and collect my thoughts on various topics here .
Check out my courses on egghead.io
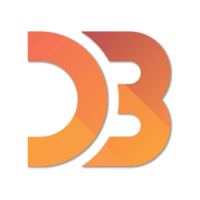
Create A Bar Chart with React and D3
D3, or Data Driven Documents, is the defacto standard for data visualization on the web. It's an incredibly powerful library that gives you all the tools you need to build just about visualization you can dream of. D3 is not a collection of predefined charts. Instead, it's a robust set of utilities you can use to ingest and transform data, to map that data to screen values, and ultimately to manipulate the DOM to render your visuals. When working with React, D3's desire to manipulate the DOM is at odds with React's goal of rendering the UI as a function of state. So how do we handle this? Simply put, we use D3 for everything up to the point where we want to render our output, and then we hand all that data to React for rendering. In this course, we'll start from scratch and build out an SVG bar chart for some sample data. We'll lean on D3 for its tools and then hand that data off to React for rendering.
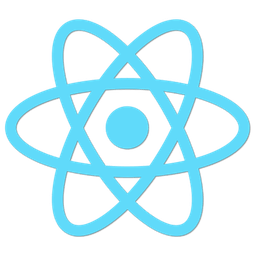
Build a React App with the Hooks API
In this collection, we build a minimal flashcard application using React, primarily focusing on function components and the hooks API. To make sure we can understand and maintain existing code, there are also a few lessons toward the end that use class components so you can get familiar with the syntax. We cover important React concepts, and sprinkle in libraries that are pretty standard in the ecosystem like @reach/router, Jest, and React testing library. We start with create-react-app so we can get right into it without worrying about configuring tooling.
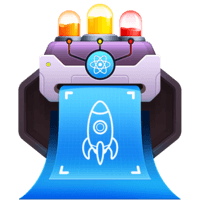
Modern JavaScript Tooling with React
Building an application with React typically involves several tools. Whether you configure these on your own or use a tool meant to save you from the configuration like Create React App, the tools are still there. When you come up against a situation where you need to start from scratch or make changes to a webpack or babel configuration, it helps to understand what each tool does, how they fit together, and how they are configured. In this course, we'll start from an empty directory and by the end, we will have configured a slim but powerful boilerplate project. Along the way, we'll touch on each of the major tools and build up to the final setup to gain an understanding of each tool's purpose and how they all work together to help you build and deliver an application.
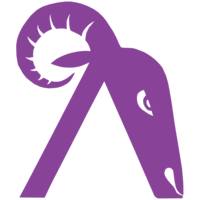
Functional Programming in JavaScript with Ramda.js
Learn how you can use ramda.js to bring functional programming concepts into your JavaScript code. Ramda offers composability and immutability right out of the box, so you can leave your imperative code behind and build cleaner, more maintainable code.
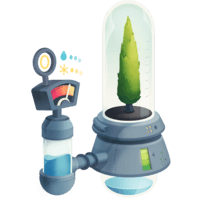
End to End testing with Cypress
The tools available for unit testing have come a long way. While testing may never be “easy”, the available tools have certainly moved testing closer to the simple end of the spectrum. Full end to end testing hasn't quite kept the same pace and as a result many applications are testing manually and often by users in production. Cypress provides a testing environment that makes end-to-end testing fast and reliable with a simple API that makes creating automated unit tests for your web applications a no-brainer.
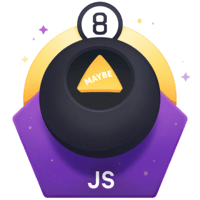
Safer JavaScript with the Maybe Type
JavaScript’s dynamic typing makes it incredibly flexible. That flexibility can lead to trouble though. When values have the potential to change types or to end up as null or undefined, that can lead to runtime errors in our code or bizarre bugs that take forever to track down because of type coercion. To battle this, we end up with code that is littered will conditionals for null or undefined values and type checks, making the core logic harder to read and refactor later. The Maybe encapsulates the type checking and guards against missing values for us. With Maybe in our toolbelt, we can keep our functions free of all the guardrails, outsource that work to the Maybe and keep our business logic free of all the clutter.
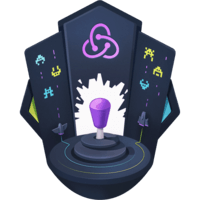
Reduce Redux Boilerplate with Redux-Actions
Redux is a powerful way to manage application state, but that power can come with a lot of code. A single update can sometimes require changes across many files and the code required to define action creators and to handle those actions in your reducer can start to get pretty repetitive. The redux-actions library offers a small but powerful API to help cut down on some of the boilerplate that is typical of an application that uses Redux to handle state management.
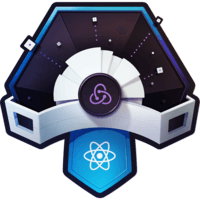
Build A React App With Redux
In this course you will learn how to build a production quality React application using Redux. We will build up from using redux by itself, so we can understand the core API and how that interacts with a React application, then we'll move on to introduce react-redux to abstract away some of the underlying details and clean up our code. We'll use middleware and a mocked API server to understand how asynchronous code fits into the Redux model and we'll even deploy our finished work so we can see it running live in the cloud.
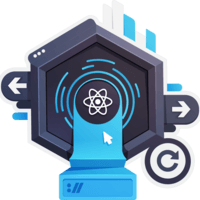
Build Your First Production Quality React App
In this course you will learn how to build production quality React applications. We will strive to keep things as simple as possible, with minimal dependencies. Instead of typing npm install, we will build our own simple solutions first, to get a solid understanding of the problems that we are solving through libraries. We will build our tools in a functional style, and write appropriate unit tests to verify that they work as expected.